Separate frontend and backend teams
You might have different frontend and backend teams that maintain their own repositories. With Amplify Gen 2, you can deploy repositories that have backend-only code, so frontend and backend teams can operate independently of each other.
Deploy the backend app
-
Run
mkdir backend-app && cd backend-app && npm create amplify@latest
to set up a backend-only Amplify project. Commit the code to a Git provider of your choice. -
Connect the
backend-app
in the new console. Navigate to the Amplify console and select Create new app. -
When you connect the repository, notice the only auto-detected framework is Amplify.
- Once you choose Save and deploy, your backend project will build.
Deploy the frontend app
- Now let's set up the frontend app and connect to the deployed backend.
npm create next-app@14 -- multi-repo-example --typescript --eslint --no-app --no-src-dir --no-tailwind --import-alias '@/*'
- Install Amplify dependencies.
cd multi-repo-examplenpm add @aws-amplify/backend-cli aws-amplify @aws-amplify/ui-react
- To connect to the deployed backend, run the following command. To locate the
App ID
for your backend application, navigate to the Amplify console and select your backend-app. On the Overview page, theApp ID
is displayed under the project name.
npx ampx generate outputs --branch main --app-id <your-backend-app-id>
This will generate the amplify_outputs.json
file that contains all the information about your backend at the root of your project.
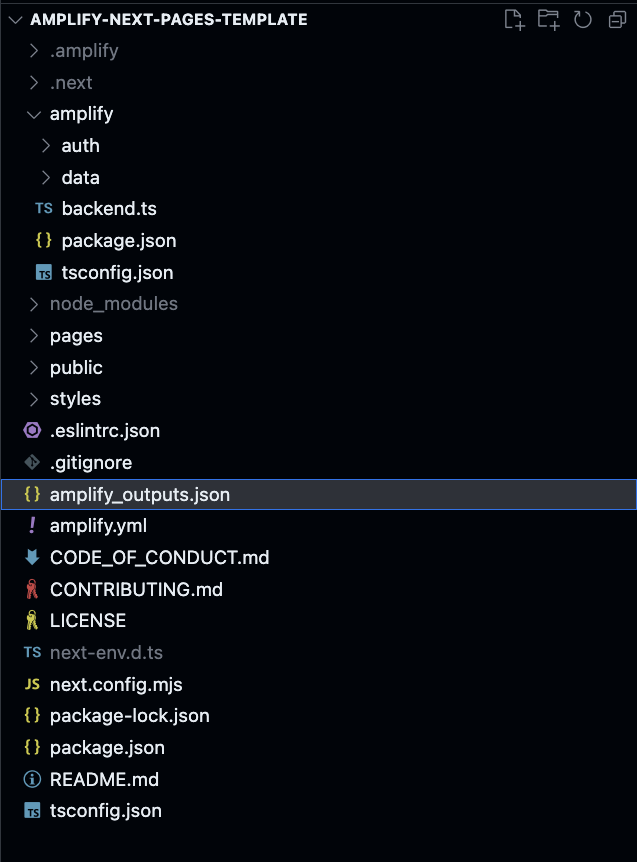
- To validate that your frontend can connect to the backend, add the
Authenticator
login form to your app.
1import { withAuthenticator } from '@aws-amplify/ui-react';2import { Amplify } from 'aws-amplify';3import outputs from '@/amplify_outputs.json';4import '@aws-amplify/ui-react/styles.css';5import '@/styles/globals.css';6import type { AppProps } from 'next/app';7
8// configure the Amplify client library with the configuration generated by `ampx sandbox`9Amplify.configure(outputs);10
11function App({ Component, pageProps }: AppProps) {12 return <Component {...pageProps} />;13}14
15export default withAuthenticator(App);
- Let's also add an
amplify.yml
build-spec to our repository.
1version: 12backend:3 phases:4 build:5 commands:6 - npm ci --cache .npm --prefer-offline7 - npx ampx generate outputs --branch main --app-id BACKEND-APPID8frontend:9 phases:10 build:11 commands:12 - npm run build13 artifacts:14 baseDirectory: .next15 files:16 - '**/*'17 cache:18 paths:19 - .next/cache/**/*20 - .npm/**/*21 - node_modules/**/*
- Now let's deploy the app. In the Amplify console, choose Create new app. Connect the repository with the default settings. You should see that the build generates the output and does not deploy a frontend. Validate that your app is working fine.
Trigger a frontend build on backend updates
The ideal scenario is that the frontend automatically retrieves the latest updates from the backend every time there is a modification made to the backend code.
-
Use the Amplify Console to create an incoming webhook.
-
Navigate to the multi-repo-example app, under Hosting > Build settings select Create webhook. Provide a name for the webhook and select the target branch to build on incoming webhook requests.
- Next, select the webhook and copy the
curl
command which will be used to trigger a build for the multi-repo-example app.
- Now update the build settings for the
backend-app
to include thecurl
command to trigger a frontend build any time there are changes to the backend.
1version: 12backend:3 phases:4 build:5 commands:6 - npm ci --cache .npm --prefer-offline7 - npx ampx pipeline-deploy --branch $AWS_BRANCH --app-id $AWS_APP_ID8frontend:9 phases:10 build:11 commands:12 - mkdir ./dist && touch ./dist/index.html13 - curl -X POST -d {} "https://webhooks.amplify.ca-central-1.amazonaws.com/prod/webhooks?id=WEBHOOK-ID&token=TOKEN&operation=startbuild" -H "Content-Type:application/json"14 artifacts:15 baseDirectory: dist16 files:17 - '**/*'18 cache:19 paths:20 - node_modules/**/*